本文作者: jsweibo
本文链接: https://jsweibo.github.io/2019/07/06/webpack%E4%B8%AD%E7%9A%84html-webpack-plugin/
摘要
本文主要讲述了:
- 如何让浏览器执行 JavaScript 文件
- webpack 中的 JavaScript 文件
- html-webpack-plugin
正文
如何让浏览器执行 JavaScript 文件
把 JavaScript 文件上传到服务器,然后再通过 URL 访问 JavaScript 文件,此时浏览器只会显示文本,但并不会执行它。
要想让浏览器执行 JavaScript 文件,必须通过<script>
将 JavaScript 注入 HTML 文件。
然后通过 URL 访问 HTML 文件的地址,此时浏览器才会执行 JavaScript 文件。
示例:
learn_js/index.html
1 |
|
learn_js/index.js
1 | console.log('hello, world'); |
webpack 中的 JavaScript 文件
明确上述概念之后,我们来看webpack
中的 JavaScript 文件。
webpack
是 JavaScript 的打包器。记住:虽然 JavaScript 打包了,但本质仍旧是 JavaScript 文件。
如果要执行打包后的 JavaScript 文件,同样必须通过<script>
将其注入 HTML 文件。
示例:
learn_webapck/webpack.config.js
1 | const path = require('path'); |
learn_webpack/src/index.js
1 | console.log('hello, world'); |
运行npx webpack
会在learn_webpack/dist/
生成一个名为bundle.js
的文件。
除此之外我们还要在learn_webpack/dist/
里放入一个 HTML。
learn_webpack/dist/index.html
1 |
|
打包完成之后把dist/
上传到服务器,通过 URL 访问 HTML 文件即可执行打包后的 JavaScript 文件。
但这种方式有许多缺点:
- 需要在
dist/
中创建一个 HTML 文件。dist/
作为出口,是不适合加入版本管理的。 - 如果修改了
output.filename
或者output.filename
以文件的 hash 值为文件名,每次打包后都要手动修改 HTML 文件。
能不能自动生成这个 HTML 文件呢?现在是html-webpack-plugin
出场的时候了。
html-webpack-plugin
安装
1 |
|
作用
简化 HTML 文件的创建。
注意:
如果使用mini-css-extract-plugin
将 JavaScript 中的 CSS 剥离成独立的文件,此时html-webpack-plugin
也会在 HTML 中使用<link>
引入剥离出来的 CSS 文件。
这和style-loader/url
不同,html-webpack-plugin
直接在静态 HTML 文件中通过<link>
注入 CSS 文件。而style-loader/url
则是通过 JavaScript 生成<link>
而后注入 CSS 文件。
示例:使用默认模板
learn_webapck/webpack.config.js
1 | const path = require('path'); |
示例:使用underscore
模板
learn_webapck/webpack.config.js
1 | const path = require('path'); |
learn_webpack/public/index.html
1 |
|
注意:
- 若要使用其他模板,例如:
handlebars
,则必须自行配置相应的 loader - 若模板已被其他 loader 处理过,则
html-webpack-plugin
将不再处理它
示例:
html-webpack-plugin
将参数交给了handlebars-loader
,handlebars-loader
支持变量注入,故仍能正常解析。由于模板已被
handlebars-loader
处理过,故html-webpack-plugin
将不再处理模板。
learn_webapck/webpack.config.js
1 | const path = require('path'); |
learn_webpack/public/index.hbs
1 |
|
示例:
html-webpack-plugin
将参数交给了html-loader
,html-loader
不支持变量注入,故<%= htmlWebpackPlugin.options.title %>
将不会被解析。由于模板已被html-loader
处理过,故html-webpack-plugin
将不再处理模板。
1 | const path = require('path'); |
learn_webpack/public/index.html
1 |
|
参数
title
<title>
的值
favicon
图片路径
html-webpack-plugin
会将图片复制到输出目录。
示例:
1 | new HtmlWebpackPlugin({ |
filename
保存文件名
默认值为index.html
template
模板路径
chunks
块列表
在 webpack 打包多页面应用时,供 HTML 文件分配各自需要引入的 JavaScript 代码块列表
excludeChunks
排除块列表
scriptLoading
脚本加载策略
取值范围:
blocking
阻塞defer
异步加载不阻塞,延迟顺序执行
默认值为blocking
参考资料
本文作者: jsweibo
本文链接: https://jsweibo.github.io/2019/07/06/webpack%E4%B8%AD%E7%9A%84html-webpack-plugin/
本文对你有帮助?请支持我
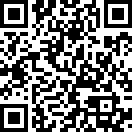
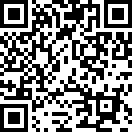
- 本文链接: https://jsweibo.github.io/2019/07/06/webpack%E4%B8%AD%E7%9A%84html-webpack-plugin/
- 版权声明: 除非另有说明,否则本网站上的内容根据署名-非商业性使用-相同方式共享 4.0 国际 (CC BY-NC-SA 4.0) 进行许可。