本文作者: jsweibo
本文链接: https://jsweibo.github.io/2019/03/14/%E5%A6%82%E4%BD%95%E5%9C%A8%E6%B5%8F%E8%A7%88%E5%99%A8%E4%B8%AD%E6%8E%A8%E9%80%81%E9%80%9A%E7%9F%A5/
摘要
本文主要讲述了:
- 什么是 Notification
- Notification.permission
- Notification.requestPermission()
- new Notification()
- 实例的
show
事件 - 实例的
close
事件 - 实例的
click
事件 - 实例的
error
事件 - Notification.prototype.close()
- 实例的 tag 属性
正文
什么是 Notification
Notification
是 1 个构造函数,它被委托在window
对象下,也可以独立使用。
开发者可以通过Notification
来推送通知。
Notification.permission
在网页向用户推送通知之前,需要向用户申请许可。
Notification.permission
表示用户许可。
取值范围:
default
未申请许可granted
同意许可denied
拒绝许可
Notification.requestPermission()
Notification.requestPermission()
用来向用户申请许可。
有 2 种调用方式。
示例:基于Promise
的调用方式
1 |
|
示例:基于callback
的调用方式
1 |
|
特别注意:对于 Chrome 62+或 Firefox 67+,只有使用 HTTPS 协议的网站才能申请许可。如果是 HTTP 协议,Notification.permission
直接变为denied
。
new Notification()
在用户同意许可之后,调用new Notification()
来构造 1 个通知对象。
示例:
1 |
|
实例的show
事件
此事件在通知显示的时候触发。
示例:
1 |
|
实例的close
事件
此事件在通知被关闭的时候触发。
注意:此事件并不一定是用户关闭触发的,也可能是通知长时间没有得到用户响应而被系统关闭触发的。
示例:
1 |
|
实例的click
事件
此事件在通知被点击的时候触发。
注意:通知在被点击之后也会触发close
事件。
示例:
1 |
|
实例的error
事件
此事件在通知发生错误的时候触发。
示例:
1 |
|
Notification.prototype.close()
此方法用来手动关闭通知。
示例:
1 |
|
实例的 tag 属性
给通知设定 1 个tag
,如果以相同的tag
的再次发起通知,就能顶替之前的通知。
示例:
1 |
|
参考资料
本文作者: jsweibo
本文链接: https://jsweibo.github.io/2019/03/14/%E5%A6%82%E4%BD%95%E5%9C%A8%E6%B5%8F%E8%A7%88%E5%99%A8%E4%B8%AD%E6%8E%A8%E9%80%81%E9%80%9A%E7%9F%A5/
本文对你有帮助?请支持我
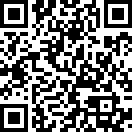
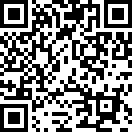