本文作者: jsweibo
本文链接: https://jsweibo.github.io/2020/06/15/JavaScript%E4%B8%AD%E7%9A%84HTMLCollection/
摘要
本文主要讲述了:
- 什么是
HTMLCollection
- 作用
- 实例属性
- 原型方法
正文
什么是HTMLCollection
一个类数组对象,原型为Object.prototype
1 |
|
作用
表示 HTML 元素集合,作为document.getElementsByClassName()
、document.getElementsByTagName()
、ParentNode.children
、document.forms
、document.scripts
、document.links
等的返回值类型
示例:
1 |
|
注意:HTMLCollection
是动态的,当 DOM 发生变更的时候,它也会自动更新
示例:动态的HTMLCollection
1 |
|
实例属性
length
实例中 HTML 元素的个数
注意:该属性为可枚举属性,这意味着你不应该使用for...in
遍历HTMLCollection
实例的属性名称
示例:
1 |
|
如果需要遍历HTMLCollection
实例中的 HTML 元素,可以使用Array.prototype.forEach
或for...of
示例:
1 |
|
原型方法
item
用于获取HTMLCollection
实例中指定下标的元素,可以使用[]
替代
示例:
1 |
|
注意:该属性为可枚举属性,这意味着你不应该使用for...in
遍历HTMLCollection
实例的属性名称
namedItem
用于获取HTMLCollection
实例中指定name
或id
的元素
示例:获取name=test
的元素
1 |
|
示例:获取id=test
的元素
1 |
|
注意:该属性为可枚举属性,这意味着你不应该使用for...in
遍历HTMLCollection
实例的属性名称
参考资料
本文作者: jsweibo
本文链接: https://jsweibo.github.io/2020/06/15/JavaScript%E4%B8%AD%E7%9A%84HTMLCollection/
本文对你有帮助?请支持我
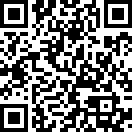
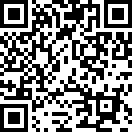
- 本文链接: https://jsweibo.github.io/2020/06/15/JavaScript%E4%B8%AD%E7%9A%84HTMLCollection/
- 版权声明: 除非另有说明,否则本网站上的内容根据署名-非商业性使用-相同方式共享 4.0 国际 (CC BY-NC-SA 4.0) 进行许可。